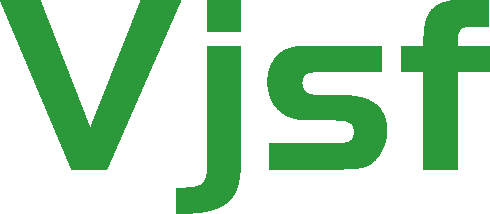
Vue JSON Schema Form
Quickly building HTML form based on Vue and JSON Schema
# Experience quickly
- Live playground (opens new window)
- Document (opens new window)
- Github (opens new window)
- Usage scenario - visual activity editor (opens new window)
- Partial plan and update plans are not supported
# NPM
npm install --save @lljj/vue-json-schema-form
# Yarn
yarn add @lljj/vue-json-schema-form
<template>
<VueForm
v-model="formData"
:ui-schema="uiSchema"
:schema="schema"
>
</VueForm>
</template>
<script >
import VueForm from '@lljj/vue-json-schema-form';
export default {
name: 'Demo',
components: {
VueForm
},
data() {
return {
formData: {},
schema: {
type: 'object',
required: [
'userName',
'age',
],
properties: {
userName: {
type: 'string',
title: 'Username',
default: 'Liu.Jun',
},
age: {
type: 'number',
title: 'Age'
},
bio: {
type: 'string',
title: 'Bio',
minLength: 10,
default: 'The more you know, the less you know',
}
}
},
uiSchema: {
bio: {
'ui:options': {
placeholder: 'Please enter your bio.',
type: 'textarea',
rows: 1
}
}
}
};
}
};
</script>
# DEMO
# Relevant
JSON Schema (opens new window) | Vue (opens new window) | Element Ui (opens new window)
# Why
Originated from shop decoration scenes, it can also be called front-end visual editing. In order to solve the universality of the component data configuration form, the form is generated through JsonSchema
.
The advantage of this is to solve the repetitive work of each configuration form, and the server can also maintain the same verification rules as the front-end based on the same schema.